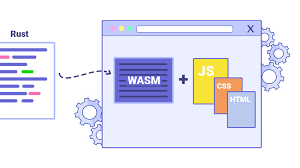
Rust WebAssembly: Building High-Performance Web Applications
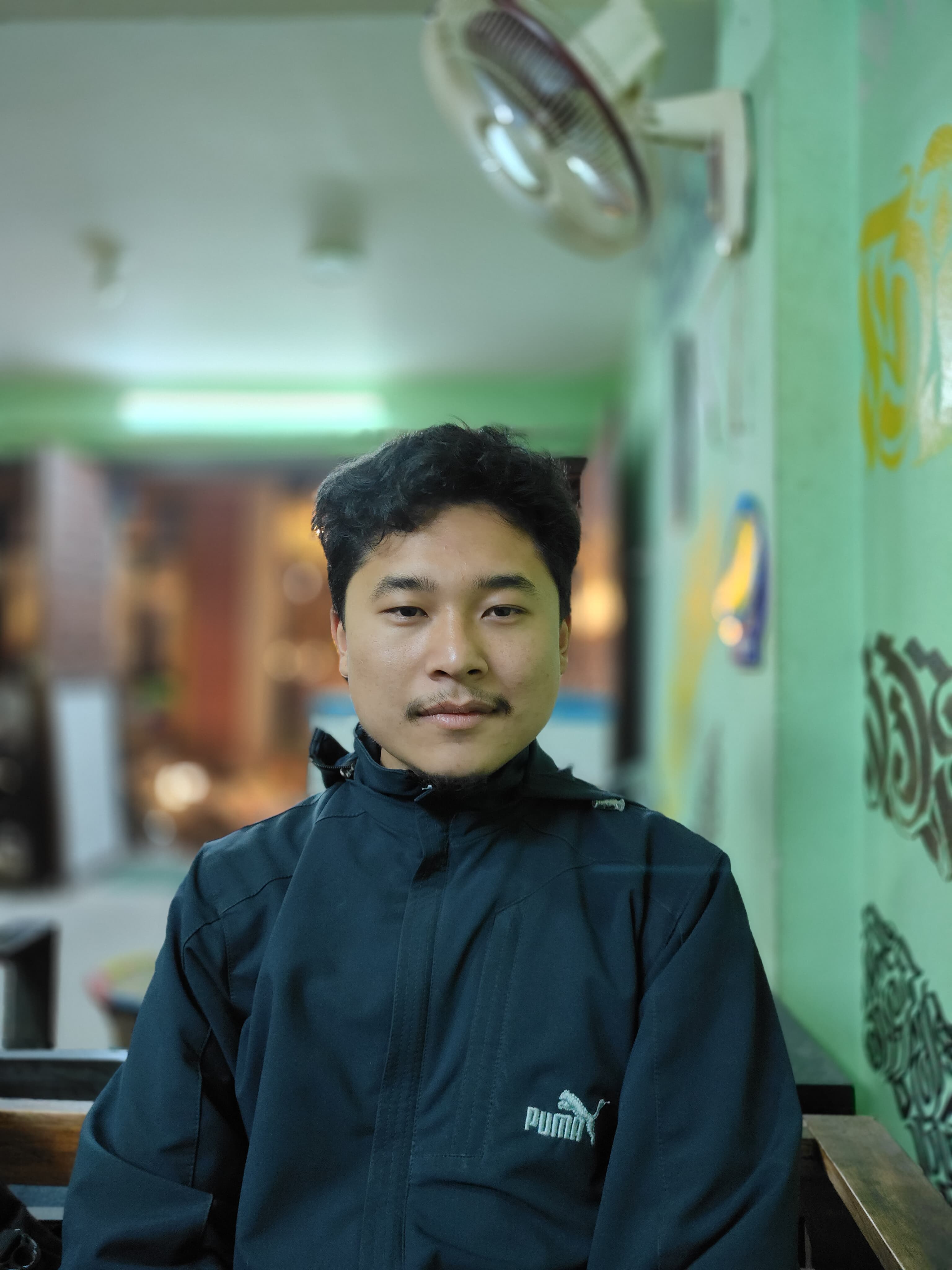
Gaurav Darlami
IoT Developer
Rust and WebAssembly (Wasm) together create a powerful combination for building high-performance web applications. Let's explore how to leverage this technology stack.
Understanding Rust and WebAssembly
WebAssembly is a binary instruction format that enables high-performance execution in web browsers. When combined with Rust, it offers: - Near-native performance - Memory safety - Type safety - Cross-platform compatibility
Getting Started
- Development Environment
Install wasm-pack cargo install wasm-pack
Install wasm-bindgen cargo install wasm-bindgen-cli ```
- Project Setup
Configure Cargo.toml ```
- Cargo.toml Configuration
[lib] crate-type = ["cdylib"]
[dependencies] wasm-bindgen = "0.2" js-sys = "0.3" web-sys = { version = "0.3", features = ["console"] } ```
Basic Implementation
- Rust Code
[wasm_bindgen] pub fn greet(name: &str) -> String { format!("Hello, {}!", name) }
[wasm_bindgen] pub struct Calculator { value: i32, }
[wasm_bindgen] impl Calculator { pub fn new() -> Calculator { Calculator { value: 0 } }
pub fn add(&mut self, x: i32) -> i32 { self.value += x; self.value }
pub fn get_value(&self) -> i32 { self.value } } ```
- JavaScript Integration
// Use the Rust functions document.getElementById("greet-button").onclick = () => { const name = document.getElementById("name-input").value; const greeting = wasm.greet(name); document.getElementById("greeting").textContent = greeting; };
// Use the Calculator struct const calc = wasm.Calculator.new(); calc.add(5); console.log(calc.get_value()); // 5 ```
- HTML Integration
Advanced Features
- Memory Management
[wasm_bindgen] impl MemoryManager { pub fn new(size: usize) -> MemoryManager { MemoryManager { data: vec![0; size], } }
pub fn get_data(&self) -> *const u8 { self.data.as_ptr() }
pub fn get_size(&self) -> usize { self.data.len() } } ```
- Error Handling
[wasm_bindgen] impl WasmError { pub fn message(&self) -> String { match self { WasmError::DivisionByZero => "Division by zero".to_string(), WasmError::Overflow => "Arithmetic overflow".to_string(), WasmError::InvalidInput => "Invalid input".to_string(), } } } ```
- Performance Optimization
// Fallback implementation data.iter().map(|&x| x * 2).collect() } ```
Best Practices
- Code Organization
- Performance
- Testing
#[test] fn test_calculator() { let mut calc = Calculator::new(); assert_eq!(calc.add(5), 5); assert_eq!(calc.add(3), 8); } } ```
Common Use Cases
- Image Processing
- Data Analysis
[wasm_bindgen] impl DataAnalyzer { pub fn new(data: Vec<f64>) -> DataAnalyzer { DataAnalyzer { data } }
pub fn calculate_mean(&self) -> f64 { self.data.iter().sum::<f64>() / self.data.len() as f64 } } ```
- Game Development
[wasm_bindgen] impl GameState { pub fn update(&mut self, dt: f32) { self.position.0 += self.velocity.0 * dt; self.position.1 += self.velocity.1 * dt; } } ```
Deployment
- Build Process
Serve the application python3 -m http.server ```
- Production Optimization
- Monitoring
Challenges and Solutions
- Debugging
- Cross-browser Compatibility
- Performance Optimization
Future Trends
- Technology Evolution
- Use Cases
- Ecosystem
Conclusion
Rust and WebAssembly together provide a powerful platform for building high-performance web applications. Key benefits include: - Near-native performance - Memory safety - Type safety - Cross-platform compatibility
Remember to: - Follow best practices - Optimize performance - Handle errors properly - Test thoroughly
The combination of Rust and WebAssembly is particularly well-suited for: - Performance-critical applications - Complex computations - Real-time processing - Resource-intensive tasks
As the ecosystem matures, we can expect to see more sophisticated applications and tools built with this powerful combination.